What is Categorial Data?
Data that can be categorized or grouped is called categorical data. It is a type of data in statistics that consists of categorial variables or data that is grouped, and it can be derived from observations made of qualitative data that are summarized as counts or from observations of quantitative data grouped within given intervals. Categorial data is also well-known as qualitative data.
Definition of Categorical Data
Categorical data is a type of data in statistics that stores data into groups or categories using names or labels.
Types of Categorial Data
Categorial Data is mainly divided into two main categories:
- Nominal Categorial Data
- Ordinal Categorial Data
They can be represented in pie charts and bar graphs respectively.
Nominal Data
Nominal Data is a type of data that consists of two or more categories without any specific order. They cannot be quantified that is put into any definite hierarchy. Variables without any quantitative value or order are labelled using nominal data.
Nominal Data is the simplest measure level and is considered the foundation of statistical analysis. Examples of Nominal data include hair, color, gender, race, place of residence, and college major.
Ordinal Data
Ordinal Categorial Data is a type of data that consists of categories with a natural rank order. However, the difference between the ranks may not be equal. It is a statistical type of quantitative data where variables exist in naturally occurring ordered categories.
Ordinal Data is used in social science and survey research, as it is relatively convenient for respondents to choose even when the underlying attribute is difficult to measure. This type of data can be easily represented using Bar Graphs, Histograms, Pie Charts, etc.
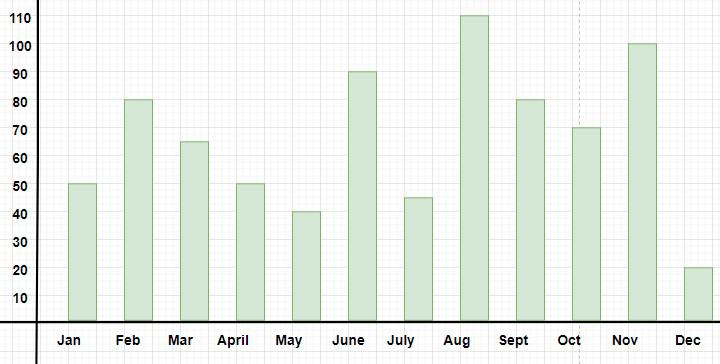
Difference Between Ordinal Data and Nominal Data
On the basis of characterstics of or ordinal data and nominal data, they can be differentiated as:
Ordinal Data Vs Nominal Data
|
Characterstics
|
Ordinal Data
|
Nominal Data
|
Definition
|
Represents categories with a specific order or ranking.
|
Represents categories with no inherent order or ranking.
|
Numeric Value
|
Grades (A, B, C), Likert scales (1st, 2nd, 3rd), Socio-economic status (Low, Medium, High).
|
Colors (Red, Blue, Green), Gender (Male, Female), Types of fruit (Apple, Orange, Banana).
|
Arithmetic Operations
|
Values have a meaningful order or sequence.
|
Values do not have a meaningful order or sequence.
|
Scale of Measurement
|
Limited arithmetic operations (e.g., you can say B is higher than C, but not by how much).
|
No meaningful arithmetic operations (e.g., no sense in saying Red + Blue = Green).
|
Examples
|
Falls under the ordinal scale.
|
Falls under the nominal scale.
|
Examples in Everyday Life
|
Ranking your preferences, ordering items by importance.
|
Categorizing items without any inherent order, like classifying colors or gender |
Features of Categorical Data
Understanding the features of categorial data can help to choose appropriate statistical methods and make meaningful interpretations.
Here are some key features of Categorial Data:
Categorial Data
Categorial data is further sub-classified into nominal and ordinal Data.
Nominal Data: Nominal data represents unordered categories or categories without any inherent order.
- Example: Colors, gender, and types of animals.
Ordinal Data: Ordinal Data represents ordered categories or categories having systematic order or ranking.
- Example: Education level (high school, college, graduate).
Mutually Exclusive
The categorial data are mutually exclusive as each observation falls into exactly one category, and no overlapping happens between categories.
Countable Categories
The categories in the categorial data are countable and distinct. They are used in frequency distribution and bar charts.
No Arithmetic Operations
The arithmetic operations are not meaningful in categorial data as you cannot perform operations like the average of categories.
Mode as Measure of Central Tendency
In categorial data, the mode is often used to describe the central tendency. It represents the most number of times a category has occurred.
Chi-Square Test
One famous statistical test for categorical data analysis is the chi-square test. It helps to determine the significant associations between two categorical variables.
Examples of Categorical Data
Some examples of categorical data are,
Pet Preference: This is an example of nominal data, where the categories are based on qualitative characteristics. The categories are dogs, cats, birds, etc.
Yes/No Questions: This is an example of binary data, where the categories are limited to two values. For example, a survey question asking if someone has a pet or not.
Color Grouping: This is an example of nominal data, where the categories are based on qualitative characteristics. The categories are red, blue, green, etc.
Breed or Model: This is an example of nominal data, where the categories are based on qualitative characteristics. The categories are poodle, bulldog, sedan, SUV, etc.
Gender: This is an example of nominal data, where the categories are based on qualitative characteristics. The categories are male, female, non-binary, etc.
Hometown: This is an example of nominal data, where the categories are based on qualitative characteristics. The categories are New York, Los Angeles, Chicago, etc.
Coffee Preference: This is an example of nominal data, where the categories are based on qualitative characteristics. The categories are latte, espresso, cappuccino, etc.
Clothing Sizes: This is an example of ordinal data, where the categories have a natural order. The categories are small, medium, large, etc.
Analysis of Categorical Data
Analysis of categorial data refers to using statistical methods to analyze data grouped into categories. These categories can be nominal (with no inherent order, like hair color) or ordinal (with an inherent order, like education level). The goal of categorial data analysis is to uncover the patterns, relationships, and insights within this data type.
Here are some common ways of analysis of Categorial Data:
Frequency Tables: Create tables to display the data counts or frequencies of different categories.
Crosstabulation: Crosstabulation of two categorical variables is performed to explore the relationship between the two variables.
Chi-Squared Tests: A statistical method used to determine if there is a significant association between two categorical variables.
Contingency Tables: Constructing a two-way table showcases the frequency of occurrence of all unique pairs of values in two columns of attribute data.
Bar Charts and Pie Charts: Categorical data's Graphical representations help visualize the categories' distribution.
Odd Ratios: It is a statistical measure used to quantify the association between two categorical variables in case-control studies.
Logistic Regression: A regression analysis used to model the relationship between a categorical dependent variable and one or more categorical or continuous independent variables.
Multiple Correspondence Analysis: A technique used to analyze the relationships among categories of multiple nominal variables.
Analysis of Variance (ANOVA): A set of statistical tests used to compare the means of three or more groups, allowing for the analysis of the effects of categorical variables on continuous outcomes.
Regression Analysis: Modeling the relationship between a continuous outcome and one or more categorical predictors, providing insights into the effects of categorical variables on continuous outcomes.
What is Categorial Variable?
A categorical variable is a type of variable in statistics that can take on a limited or usually fixed number of possible values, assigning each individual or other unit of observation to a particular group or nominal category on the basis of a characteristic.
- Qualitative variables or attribute variables are other names for categorical variables. They may be ordinal or nominal.
- Nominal variables describe a name, label, or category without natural order.
In contrast, ordinal variables have a straightforward ordering of the categories. Examples of categorical variables include demographic information of a population, college major, and the roll of a six-sided die.
Advantages of Categorical Data
The advantages below show the value of categorical data for various analytical and business purposes, including market segmentation, trend analysis, and targeted marketing. The following are the advantages of categorical data:
- Easy Interpretation: Categorial data is easier to interpret and analyze than quantitative data, making it an ideal choice for individuals without a strong background in mathematics or statistics.
- Quick Recognition of Trends and Patterns: Categorical data allows for the quick recognition of trends, changes, and patterns based on interrelated variables, making the information easier to digest and understand
- Segmentation for Targeted Marketing: The segmentation of categorial data helps to differentiate customers into different groups for targeted marketing, allowing businesses to modify their strategies to specific customer segmentation.
- Use in Correlation and Trend Analysis: Categorial data is beneficial in understanding how different populations interact with each other, as well as for ascertaining correlations between different variables and understanding trends and patterns within a population.
- Concrete Results: The results of categorical data are concrete, without subjective, open-ended questions, providing straightforward insights.
Disadvantages of Categorical Data
There are some disadvantages to using categorical data, which are mentioned below:
- Limited Statistical Analysis: Categorical data is limited to the kind of statistical analysis that can be performed on it. It does not have the same statistical properties as quantitative data, which means that numerical or statistical analysis cannot be performed on categorical data.
- Loss of Detail: When continuous variables are categorized, a level of detail is lost. This can make it challenging to analyze the data and may result in a less accurate representation of the underlying patterns or relationships.
- Low Sensitivity: Categorical data research is often low in sensitivity, with responses typically being either good/bad or yes/no. This can limit the ability to detect subtle differences or trends in the data.
- Expensive and Time-Consuming: Categorical data requires larger samples, which can be more expensive and time-consuming to gather compared to quantitative data.
- Potential for Irrelevant Data: When collecting categorical data, researchers may have to handle irrelevant data, which can add complexity to the data analysis process
Categorical and Numerical Data
On the basis of aspects of categorical data and nominal data, they can be differentiated as:
Categorical Data Vs Nominal Data
|
Aspects
|
Categorial Data
|
Numerical Data
|
Other Name
|
Qualitative Data
|
Quantitative Data
|
Nature of Data
|
Non-numerical and can be identified based on names or labels
|
Form of numbers and can be used for arithmetic processes.
|
Types Of Data
|
Nominal and Ordinal Data
|
Discrete and Continuous Data
|
Analysis Technique
|
Perform research involving qualitative analysis
|
Perform calculation problem in statistics.
|
Examples
|
Name, Gender, Phone Number etc.
|
Measurement, Such as height and weight, etc.
|
Application Of Categorial Data
Categorial data is divided into nominal and ordinal Data. They have various real-world applications. Here are some of the real-world examples of them.
Nominal Data is used in places such as purchase information, where non-numerical, unordered categorical data is collected from customers for activities like shipping orders or serving food is considered nominal.
Educational levels, income ranges, and customer satisfaction surveys all lie in ordinal data, where data has a natural order or ranking.
Challenges In Categorial Data
While working with categorial data, several challenges need to be considered. Some of these challenges include:
- Data Quality: Ensuring the accuracy and consistency of categorical data is crucial for accurate analysis. Errors in categorization or incorrect labelling can lead to incorrect insights and conclusions.
- Measurement Error: Ordinal data with a ranked order can suffer from measurement error due to the lack of consistent spacing between ranks. This can make it difficult to compare and analyze the data accurately.
- Mutually Exclusive Categories: Categories in categorical data must be mutually exclusive, meaning each category should not overlap with any other category. This ensures that the data is properly organized and can be analyzed effectively.
- Lack of Quantitative Information: Nominal data does not provide any quantitative information, which can limit the types of analyses and insights that can be derived from the data.
- Difficulty in Ranking: Nominal data cannot be ranked or ordered, making it challenging to compare and analyze the data in a meaningful way.
- Limited Analysis Options: Nominal data has fewer analysis options compared to ordinal data, as it does not provide any information about the ranking or order of the categories.
- Handling Irrelevant Data: Nominal data, which is often collected through surveys or questionnaires, can sometimes contain irrelevant or empty responses. Researchers need to find ways to handle this irrelevant data to ensure accurate analysis.
Examples on Categorical Data
Example 1: Favorite Ice Cream Flavors
You conduct a survey in your school cafeteria to find out students' favorite ice cream flavors. You collect the following data:
Student
|
Favorite Flavor
|
John
|
Chocolate
|
Mary
|
Vanilla
|
Peter
|
Mint Chocolate Chip
|
Alice
|
Chocolate
|
Bob
|
Strawberry
|
Sarah
|
Strawberry
|
Solution:
Data is categorical because "Favorite Flavor" has distinct categories like chocolate, strawberry, vanilla, etc. You can analyze this data in various ways below is one such way:
Create a table showing the number of students who prefer each flavor.
Flavor
|
Frequency
|
Chocolate
|
2
|
Strawberry
|
2
|
Vanilla
|
1
|
Mint Chocolate Chip
|
1
|
Example 2: Movie Genre Preferences
You ask your classmates about their favorite movie genres and get the following data:
Students
|
Favorite Genre
|
David
|
Animation
|
Emma
|
Sci-Fi
|
Liam
|
Action
|
Olivia
|
Drama
|
Adam
|
Comedy
|
Noah
|
Comedy
|
Solution:
Data is categorical because "Favorite Genre" has distinct categories like Comedy, Drama, Action, etc.
Create a table showing the number of students who prefer each Genre.
Genre
|
Frequency
|
Comedy
|
2
|
Action
|
1
|
Drama
|
1
|
Sci-Fi
|
1
|
Animation
|
1
|
You can represent this both example in Pie chart as well as in Bar graph.
Example 3: Clothing Sizes
You ask a group of people their clothing size, and you get the following responses:
Person 1: Medium
Person 2: Large
Person 3: Small
Person 4: Medium
Person 5: Large
Solution:
Create a frequency table:
Size
|
Frequency
|
Small
|
1
|
Medium
|
2
|
Large
|
2
|
Example 4: Color Preference Survey
You survey a group of people to find out their favorite colors:
Person 1: Blue
Person 2: Red
Person 3: Green
Person 4: Blue
Person 5: Red
Solution:
Create a frequency table:
Color
|
Frequency
|
Blue
|
2
|
Red
|
2
|
Green
|
1
|
Example 5: Students’ Sports Participation
You collect data on sports participation in your school:
Alex: Basketball, Soccer
Ben: Baseball
Emma: Volleyball
Chloe: Tennis, Swimming
David: Basketball, Track
Solution:
This data is categorical, and you can create a frequency table showing the number of students participating in each sport:
Sport
|
Frequency
|
Basketball
|
2
|
Soccer
|
1
|
Baseball
|
1
|
Volleyball
|
1
|
Tennis
|
1
|
Swimming
|
1
|
Track
|
1
|
Practice Questions on Categorical Data
1: Sports Survey: Your school wants to improve its sports program and asks students which sports they participate in. You collect the following data:
Student
|
Sports
|
Alex
|
Basketball, Soccer
|
Ben
|
Baseball
|
Emma
|
Volleyball
|
Chloe
|
Tennis, Swimming
|
David
|
Basketball, Track
|
- Create a frequency table showing the number of students who participate in each sport.
- Draw a bar chart to visualize the popularity of different sports.
- If your school has budget limitations, which sports might be prioritized based on this data? Why?
2: You want to understand your classmates' lunch habits. You ask them about their preferred lunch options (packed lunch, school cafeteria, fast food) and their grade level. Examine the data and answer these questions:
- Are there any differences in lunch preferences between different grade levels? Explain your findings.
- If you were in charge of the school cafeteria, what changes might you make based on this data?
3: A teacher wants to know how students travel to school. The options are Bus, Car, Bike, Walk. Create a frequency table and visualize the data using a bar chart.
4: Survey the favorite social media platform (Facebook, Instagram, Twitter, LinkedIn) of 10 people. Create a frequency table and visualize the data using a pie chart.
5: You collect data on the favorite pet animals (Dog, Cat, Fish, Bird) among 20 people. Create a frequency table and represent it using a bar chart.
6: A restaurant owner wants to know the preferred payment methods (Cash, Card, Mobile Payment) of their customers. Analyze the data by creating a frequency table and visualizing it with a pie chart.
7: A school survey asks students about their favorite subject (Math, Science, History, English). Create a frequency table and visualize the data using a bar chart.
8: You want to find out the most common shoe size in your neighborhood. The options are 7, 8, 9, 10. Create a frequency table and represent the data using a bar chart.
9: A researcher surveys people on their coffee preference (Latte, Espresso, Cappuccino, Americano). Create a frequency table and visualize the data using a pie chart.
10: Conduct a survey to find out the preferred movie-watching method (Theater, Streaming, DVD) among a group of friends. Analyze the data by creating a frequency table and visualizing it with a bar chart.
Authors: T. C. Okenna, GeeksforGeeks
Register for this course: Enrol Now